大家好,欢迎来到IT知识分享网。
此文,仅做为个人学习Android,记录成长以及方便复习!
1.配置一下界面,使用RadioGroup实现底部导航栏,其中的4个按钮,本次是只使用传值通讯一个按钮!
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" > <LinearLayout android:id="@+id/linearlayout" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal"></LinearLayout> <RadioGroup android:id="@+id/radiogrop" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:orientation="horizontal"> <RadioButton android:id="@+id/one" android:background="@drawable/radio_pressed" android:drawableTop="@drawable/ic_launcher_foreground" android:gravity="center_horizontal" android:text="静态加载" android:button="@null" android:layout_width="0dp" android:layout_weight="1" android:layout_height="wrap_content"/> <RadioButton android:id="@+id/two" android:background="@drawable/radio_pressed" android:drawableTop="@drawable/ic_launcher_foreground" android:gravity="center_horizontal" android:text="动态加载" android:button="@null" android:layout_width="0dp" android:layout_weight="1" android:layout_height="wrap_content"/> <RadioButton android:id="@+id/three" android:background="@drawable/radio_pressed" android:drawableTop="@drawable/ic_launcher_foreground" android:gravity="center_horizontal" android:text="生命周期" android:button="@null" android:layout_width="0dp" android:layout_weight="1" android:layout_height="wrap_content"/> <RadioButton android:id="@+id/four" android:background="@drawable/radio_pressed" android:drawableTop="@drawable/ic_launcher_foreground" android:gravity="center_horizontal" android:text="传值通讯" android:button="@null" android:layout_width="0dp" android:layout_weight="1" android:layout_height="wrap_content"/> </RadioGroup> </RelativeLayout>
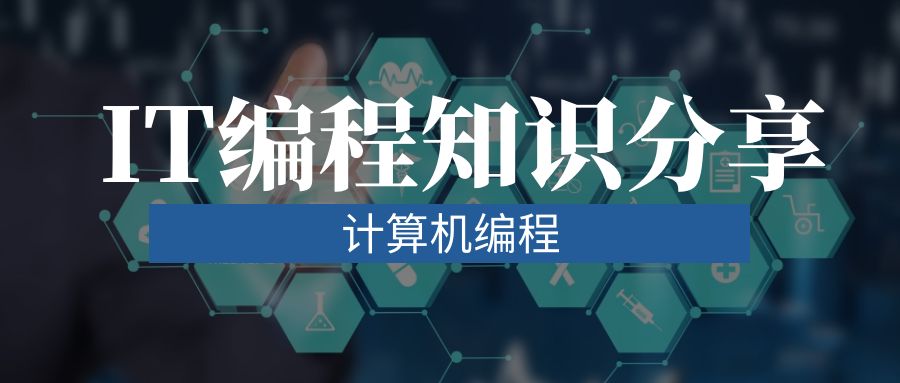
接下来是底部导航栏的选择器配置文件
radio_pressed.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@color/garay" android:state_checked="true"></item> <item android:drawable="@color/white"></item> </selector>
接下来创建一个Activity
MainActivity.java
package com.rui.fragmentdemo; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.widget.RadioGroup; public class MainActivity extends Activity{ private RadioGroup radiogroup;//声明RadioGroup @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //实例化RadioGroup radiogroup = (RadioGroup)findViewById(R.id.radiogrop); //使用RadioGroup监听事件,使用监听方法OnCheckedChangeListener radiogroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() { @Override public void onCheckedChanged(RadioGroup radioGroup, int i) { switch (i){ case R.id.one: case R.id.two: case R.id.three: case R.id.four: //跳转MainActivity3 Intent intent1 = new Intent(MainActivity.this,MainActivity3.class); startActivity(intent1); break; } } }); } }
创建布局文件layout.xml,为MainActivity3提供布局
layout.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/linear" android:tag="tag" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <EditText android:id="@+id/edittext" android:layout_width="match_parent" android:layout_height="wrap_content"/> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="发送"/> </LinearLayout>
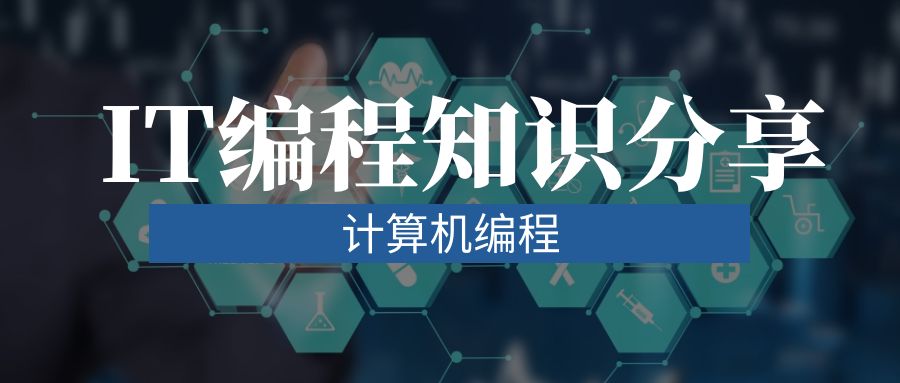
创建布局activity_main3提供Fragment转换
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/tview" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
创建一个继承Fragment的类
MyFragment2.java
package com.rui.fragmentdemo; import android.app.Activity; import android.app.Fragment; import android.os.Bundle; import android.support.annotation.Nullable; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; import android.widget.Toast; /** * Created by azl001 on 2018/3/21. */ public class MyFragment2 extends Fragment{ private String code="一个简单的小答复!";//测试回传给Acticity的值 public Listener listener;//获取一个Listener对象 //定义一个接口,里面定义一个无实体的方法,方便Activity调用 public interface Listener { public void message(String code); } //Fragment添加到Activity的时候运行 @Override public void onAttach(Activity activity) { super.onAttach(activity); //将activity赋予listener,让listerner得到Activity对象 listener = (Listener)activity; } @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, Bundle savedInstanceState) { //布局转换成View View view = inflater.inflate(R.layout.activity_main3,container,false); //通过View实例化TextView TextView tv1 = (TextView) view.findViewById(R.id.tview); //获取Activity传送过来的值 String text = getArguments().get("value")+""; //把获取的值设置到tv1中 tv1.setText(text); Toast.makeText(getActivity(),"接收到了Activity发送来的:"+text,Toast.LENGTH_SHORT).show(); Toast.makeText(getActivity(),"向Activity发送消息:"+code,Toast.LENGTH_SHORT).show(); //将消息放入message让Activity回调得到消息内容 listener.message(code); return view; } }
接下来配置MainActivity3
MainActivity3.java
package com.rui.fragmentdemo; import android.app.Activity; import android.app.FragmentManager; import android.app.FragmentTransaction; import android.os.Bundle; import android.support.annotation.Nullable; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; /** * Created by azl001 on 2018/3/21. */ public class MainActivity3 extends Activity implements MyFragment2.Listener{ private EditText editText;//声明tv1 private Button bt1;//声明bt1 @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.layout); //实例化tv1,bt1 editText = (EditText) findViewById(R.id.edittext); bt1 = (Button)findViewById(R.id.button); //按钮监听事件 bt1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //获取输入框的值 String value = editText.getText().toString(); //实例化MyFragment2对象 MyFragment2 mf2 = new MyFragment2(); //实例化一个Bundle 用来存放数据 Bundle bundle = new Bundle(); //Bundle加入编辑框数据 bundle.putString("value",value); //发送给Fragment mf2.setArguments(bundle); //获取事物管理者 FragmentManager fm = getFragmentManager(); //开启事物 FragmentTransaction ft = fm.beginTransaction(); //向fragemnt添加fragment // 参数1 是添加到哪个布局 //参数2 继承了Fragment的类 //参数3 Fragment的tag 和 id 类似 ft.add(R.id.linear,mf2,"tag"); //提交事务 ft.commit(); Toast.makeText(MainActivity3.this,"向Fragment发送数:”:"+value,Toast.LENGTH_SHORT).show(); } }); } //实现Fragment的接口方法,用于接收Fragment发送的消息 @Override public void message(String code) { Toast.makeText(MainActivity3.this,"接收到了Fragment的回复:"+code,Toast.LENGTH_SHORT).show(); } }
效果如下:
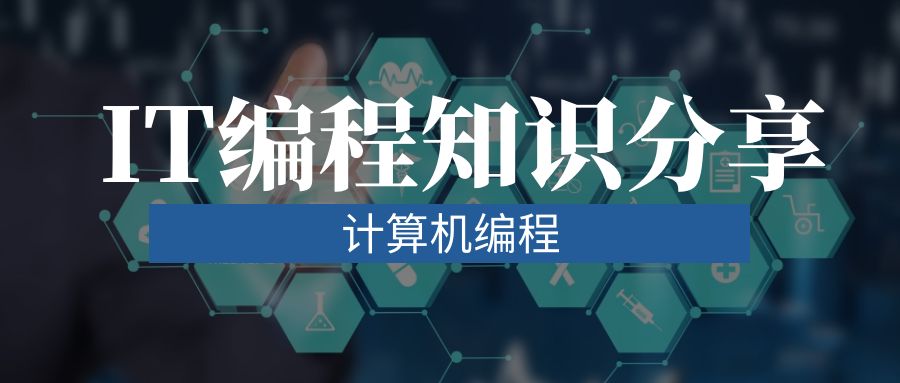
免责声明:本站所有文章内容,图片,视频等均是来源于用户投稿和互联网及文摘转载整编而成,不代表本站观点,不承担相关法律责任。其著作权各归其原作者或其出版社所有。如发现本站有涉嫌抄袭侵权/违法违规的内容,侵犯到您的权益,请在线联系站长,一经查实,本站将立刻删除。 本文来自网络,若有侵权,请联系删除,如若转载,请注明出处:https://yundeesoft.com/55399.html