大家好,欢迎来到IT知识分享网。
1、选项式Api与组合式Api
vue2:
<template> <div @click="changeMsg">{{msg}}</div> </template> <script> export default { data(){ return { msg:'hello world' } }, methods:{ changeMsg(){ this.msg = 'hello juejin' } } } </script>
vue3:
<template> <div @click="changeMsg">{{ msg }}</div> </template> <script setup> import { ref } from "vue"; const msg = ref('hello world') const changeMsg = () => { msg.value = 'hello juejin' } </script>
总结: 选项式Api是将data和methods包括后面的watch,computed等分开管理,而组合式Api则是将相关逻辑放到了一起(类似于原生js开发)。 setup语法糖则可以让变量方法不用再写return,后面的组件甚至是自定义指令也可以在我们的template中自动获得。 2、ref 和 reactive
<script setup> import { ref,reactive } from "vue"; let msg = ref('hello world') let obj = reactive({ name:'juejin', age:3 }) const changeData = () => { msg.value = 'hello juejin' obj.name = 'hello world' } </script>
总结: 使用ref的时候在js中取值的时候需要加上.value。
reactive更推荐去定义复杂的数据类型 ref 更推荐定义基本类型
3、生命周期
下表包含:Vue2和Vue3生命周期的差异
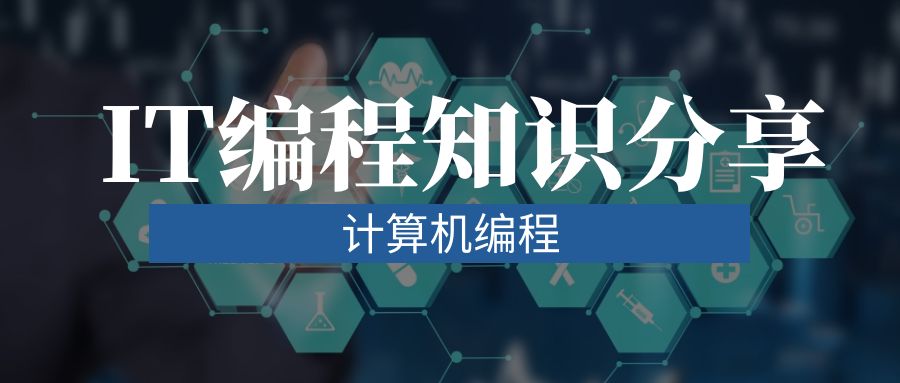
添加图片注释,不超过 140 字(可选)
vue2
<script> export default { mounted(){ console.log('挂载完成') } } </script>
vue3
<script setup> import { onMounted } from "vue"; onMounted(()=>{ console.log('挂载完成') }) </script>
从上面可以看出Vue3中的组合式API采用hook函数引入生命周期;其实不止生命周期采用hook函数引入,像watch、computed、路由守卫等都是采用hook函数实现 总结 Vue3中的生命周期相对于Vue2做了一些调整,命名上发生了一些变化并且移除了beforeCreate和created,因为setup是围绕beforeCreate和created生命周期钩子运行的,所以不再需要它们。 生命周期采用hook函数引入 4、watch和computed vue2
<template> <div>{{ addSum }}</div> </template> <script> export default { data() { return { a: 1, b: 2 } }, computed: { addSum() { return this.a + this.b } }, watch:{ a(newValue, oldValue){ console.log(`a从${oldValue}变成了${newValue}`) } } } </script>
vue3
<template> <div>{{ addSum }}</div> </template> <script setup> import { computed, ref, watch } from "vue"; const a = ref(1) const b = ref(2) const form = ref({ value: '' }) let addSum = computed(() => { return a.value + b.value }) watch(a, (newValue, oldValue) => { console.log(`a从${oldValue}变成了${newValue}`) }) watch( form, async (now, old) => { console.log(now.old,"old") }, { deep: true, }, ) </script>
Vue3中除了watch,还引入了副作用监听函数watchEffect,用过之后我发现它和React中的useEffect很像,只不过watchEffect不需要传入依赖项。 那么什么是watchEffect呢? watchEffect它会立即执行传入的一个函数,同时响应式追踪其依赖,并在其依赖变更时重新运行该函数。 比如这段代码
<template> <div>{{ watchTarget }}</div> </template> <script setup> import { watchEffect,ref } from "vue"; const watchTarget = ref(0) watchEffect(()=>{ console.log(watchTarget.value) }) setInterval(()=>{ watchTarget.value++ },1000) </script>
首先刚进入页面就会执行watchEffect中的函数打印出:0,随着定时器的运行,watchEffect监听到依赖数据的变化回调函数每隔一秒就会执行一次 总结 computed和watch所依赖的数据必须是响应式的。Vue3引入了watchEffect,watchEffect 相当于将 watch 的依赖源和回调函数合并,当任何你有用到的响应式依赖更新时,该回调函数便会重新执行。不同于 watch的是watchEffect的回调函数会被立即执行,即({ immediate: true }) 5、组件通信 Vue中组件通信方式有很多,其中选项式API和组合式API实现起来会有很多差异;这里将介绍如下组件通信 方式:
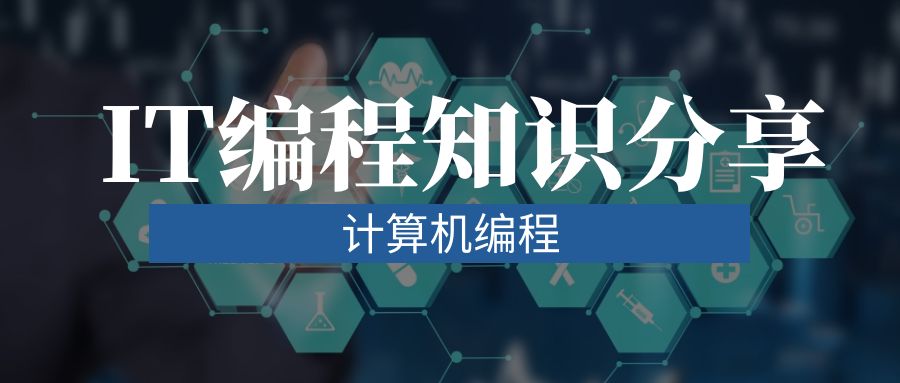
添加图片注释,不超过 140 字(可选)
props
vue2
//父组件 <template> <div> <Child :msg="parentMsg" /> </div> </template> <script> import Child from './Child' export default { components:{ Child }, data() { return { parentMsg: '父组件信息' } } } </script> //子组件 <template> <div> {{msg}} </div> </template> <script> export default { props:['msg'] } </script>
vue3
//父组件 <template> <div> <Child :msg="parentMsg" /> </div> </template> <script setup> import { ref } from 'vue' import Child from './Child.vue' const parentMsg = ref('父组件信息') </script> //子组件 <template> <div> {{ parentMsg }} </div> </template> <script setup> import { toRef, defineProps } from "vue"; const props = defineProps(["msg"]); console.log(props.msg) //父组件信息 let parentMsg = toRef(props, 'msg') </script>
注意 props中数据流是单项的,即子组件不可改变父组件传来的值 在组合式API中,如果想在子组件中用其它变量接收props的值时需要使用toRef将props中的属性转为响应式。 emit 子组件可以通过emit发布一个事件并传递一些参数,父组件通过v-on进行这个事件的监听 vue2
//父组件 <template> <div> <Child @sendMsg="getFromChild" /> </div> </template> <script> import Child from './Child' export default { components:{ Child }, methods: { getFromChild(val) { console.log(val) //我是子组件数据 } } } </script> // 子组件 <template> <div> <button @click="sendFun">send</button> </div> </template> <script> export default { methods:{ sendFun(){ this.$emit('sendMsg','我是子组件数据') } } } </script>
vue3
//父组件 <template> <div> <Child @sendMsg="getFromChild" /> </div> </template> <script setup> import Child from './Child' const getFromChild = (val) => { console.log(val) //我是子组件数据 } </script> //子组件 <template> <div> <button @click="sendFun">send</button> </div> </template> <script setup> import { defineEmits } from "vue"; const emits = defineEmits(['sendMsg']) const sendFun = () => { emits('sendMsg', '我是子组件数据') } </script>
attrs和listeners 子组件使用attrs可以获得父组件除了props传递的属性和特性绑定属性(class和style)之外的所有属性。子组件使用attrs可以获得父组件除了props传递的属性和特性绑定属性 (class和 style)之外的所有属性。 子组件使用attrs可以获得父组件除了props传递的属性和特性绑定属性(class和style)之外的所有属性。子组件使用listeners可以获得父组件(不含.native修饰器的)所有v-on事件监听器,在Vue3中已经不再使用;但是Vue3中的attrs不仅可以获得父组件传来的属性也可以获得父组件v-on事件监听器 vue2
//父组件 <template> <div> <Child @parentFun="parentFun" :msg1="msg1" :msg2="msg2" /> </div> </template> <script> import Child from './Child' export default { components:{ Child }, data(){ return { msg1:'子组件msg1', msg2:'子组件msg2' } }, methods: { parentFun(val) { console.log(`父组件方法被调用,获得子组件传值:${val}`) } } } </script> //子组件 <template> <div> <button @click="getParentFun">调用父组件方法</button> </div> </template> <script> export default { methods:{ getParentFun(){ this.$listeners.parentFun('我是子组件数据') } }, created(){ //获取父组件中所有绑定属性 console.log(this.$attrs) //{"msg1": "子组件msg1","msg2": "子组件msg2"} //获取父组件中所有绑定方法 console.log(this.$listeners) //{parentFun:f} } } </script>
vue3
//父组件 <template> <div> <Child @parentFun="parentFun" :msg1="msg1" :msg2="msg2" /> </div> </template> <script setup> import Child from './Child' import { ref } from "vue"; const msg1 = ref('子组件msg1') const msg2 = ref('子组件msg2') const parentFun = (val) => { console.log(`父组件方法被调用,获得子组件传值:${val}`) } </script> //子组件 <template> <div> <button @click="getParentFun">调用父组件方法</button> </div> </template> <script setup> import { useAttrs } from "vue"; const attrs = useAttrs() //获取父组件方法和事件 console.log(attrs) //Proxy {"msg1": "子组件msg1","msg2": "子组件msg2"} const getParentFun = () => { //调用父组件方法 attrs.onParentFun('我是子组件数据') } </script>
注意 Vue3中使用attrs调用父组件方法时,方法前需要加上on;如parentFun->onParentFun provide/inject provide:是一个对象,或者是一个返回对象的函数。里面包含要给子孙后代属性 inject:一个字符串数组,或者是一个对象。获取父组件或更高层次的组件provide的值,既在任何后代组件都可以通过inject获得 vue2
//父组件 <script> import Child from './Child' export default { components: { Child }, data() { return { msg1: '子组件msg1', msg2: '子组件msg2' } }, provide() { return { msg1: this.msg1, msg2: this.msg2 } } } </script> //子组件 <script> export default { inject:['msg1','msg2'], created(){ //获取高层级提供的属性 console.log(this.msg1) //子组件msg1 console.log(this.msg2) //子组件msg2 } } </script>
vue3
//父组件 <script setup> import Child from './Child' import { ref,provide } from "vue"; const msg1 = ref('子组件msg1') const msg2 = ref('子组件msg2') provide("msg1",msg1) provide("msg2",msg2) </script> //子组件 <script setup> import { inject } from "vue"; console.log(inject('msg1').value) //子组件msg1 console.log(inject('msg2').value) //子组件msg2 </script>
说明 provide/inject一般在深层组件嵌套中使用合适。一般在组件开发中用的居多。 parent/children $parent: 子组件获取父组件Vue实例,可以获取父组件的属性方法等 $children: 父组件获取子组件Vue实例,是一个数组,是直接儿子的集合,但并不保证子组件的顺序 vue2
import Child from './Child' export default { components: { Child }, created(){ console.log(this.$children) //[Child实例] console.log(this.$parent)//父组件实例 } }
注意 父组件获取到的$children并不是响应式的
expose&ref
$refs可以直接获取元素属性,同时也可以直接获取子组件实例
vue2
//父组件 <template> <div> <Child ref="child" /> </div> </template> <script> import Child from './Child' export default { components: { Child }, mounted(){ //获取子组件属性 console.log(this.$refs.child.msg) //子组件元素 //调用子组件方法 this.$refs.child.childFun('父组件信息') } } </script> //子组件 <template> <div> <div></div> </div> </template> <script> export default { data(){ return { msg:'子组件元素' } }, methods:{ childFun(val){ console.log(`子组件方法被调用,值${val}`) } } } </script>
vue3
//父组件 <template> <div> <Child ref="child" /> </div> </template> <script setup> import Child from './Child' import { ref, onMounted } from "vue"; const child = ref() //注意命名需要和template中ref对应 onMounted(() => { //获取子组件属性 console.log(child.value.msg) //子组件元素 //调用子组件方法 child.value.childFun('父组件信息') }) </script> //子组件 <template> <div> </div> </template> <script setup> import { ref,defineExpose } from "vue"; const msg = ref('子组件元素') const childFun = (val) => { console.log(`子组件方法被调用,值${val}`) } //必须暴露出去父组件才会获取到 defineExpose({ childFun, msg }) </script>
注意 通过ref获取子组件实例必须在页面挂载完成后才能获取。 在使用setup语法糖时候,子组件必须元素或方法暴露出去父组件才能获取到 EventBus/mitt 兄弟组件通信可以通过一个事件中心EventBus实现,既新建一个Vue实例来进行事件的监听,触发和销毁。 在Vue3中没有了EventBus兄弟组件通信,但是现在有了一个替代的方案mitt.js,原理还是 EventBus vue2
//组件1 <template> <div> <button @click="sendMsg">传值</button> </div> </template> <script> import Bus from './bus.js' export default { data(){ return { msg:'子组件元素' } }, methods:{ sendMsg(){ Bus.$emit('sendMsg','兄弟的值') } } } </script> //组件2 <template> <div> 组件2 </div> </template> <script> import Bus from './bus.js' export default { created(){ Bus.$on('sendMsg',(val)=>{ console.log(val);//兄弟的值 }) } } </script> //bus.js import Vue from "vue" export default new Vue()
vue3
首先安装mitt
npm i mitt -S
mitt.js
import mitt from 'mitt' const Mitt = mitt() export default Mitt
//组件1 <template> <button @click="sendMsg">传值</button> </template> <script setup> import Mitt from './mitt.js' const sendMsg = () => { Mitt.emit('sendMsg', '兄弟的值') } </script> //组件2 <template> <div> 组件2 </div> </template> <script setup> import { onUnmounted } from "vue"; import Mitt from './mitt.js' const getMsg = (val) => { console.log(val);//兄弟的值 } Mitt.on('sendMsg', getMsg) onUnmounted(() => { //组件销毁 移除监听 Mitt.off('sendMsg', getMsg) }) </script>
v-model和sync v-model大家都很熟悉,就是双向绑定的语法糖。这里不讨论它在input标签的使用;只是看一下它和sync在组件中的使用 我们都知道Vue中的props是单向向下绑定的;每次父组件更新时,子组件中的所有props都会刷新为最新的值;但是如果在子组件中修改 props ,Vue会向你发出一个警告(无法在子组件修改父组件传递的值);可能是为了防止子组件无意间修改了父组件的状态,来避免应用的数据流变得混乱难以理解。 但是可以在父组件使用子组件的标签上声明一个监听事件,子组件想要修改props的值时使用$emit触发事件并传入新的值,让父组件进行修改。 为了方便vue就使用了v-model和sync语法糖。 vue2
//父组件 <template> <div> <!-- 完整写法 <Child @update:changePval="msg=$event" /> --> <Child :changePval.sync="msg" /> {{msg}} </div> </template> <script> import Child from './Child' export default { components: { Child }, data(){ return { msg:'父组件值' } } } </script> //子组件 <template> <div> <button @click="changePval">改变父组件值</button> </div> </template> <script> export default { data(){ return { msg:'子组件元素' } }, methods:{ changePval(){ //点击则会修改父组件msg的值 this.$emit('update:changePval','改变后的值') } } } </script>
vue3
//父组件 <template> <div> <!-- 完整写法 <Child @update:changePval="msg=$event" /> --> <Child v-model:changePval="msg" /> {{msg}} </div> </template> <script setup> import Child from './Child' import { ref } from 'vue' const msg = ref('父组件值') </script> //子组件 <template> <button @click="changePval">改变父组件值</button> </template> <script setup> import { defineEmits } from 'vue'; const emits = defineEmits(['changePval']) const changePval = () => { //点击则会修改父组件msg的值 emits('update:changePval','改变后的值') } </script>
总结 vue3中移除了sync的写法,取而代之的式v-model:event的形式 其v-model:changePval=”msg”或者:changePval.sync=”msg”的完整写法为 @update:changePval=”msg=$event”。 所以子组件需要发送update:changePval事件进行修改父组件的值 6、路由 vue3和vue2路由常用功能只是写法上有些区别 vue2
<template> <div> <button @click="toPage">路由跳转</button> </div> </template> <script> export default { beforeRouteEnter (to, from, next) { // 在渲染该组件的对应路由被 confirm 前调用 next() }, beforeRouteEnter (to, from, next) { // 在渲染该组件的对应路由被 confirm 前调用 next() }, beforeRouteLeave ((to, from, next)=>{//离开当前的组件,触发 next() }), beforeRouteLeave((to, from, next)=>{//离开当前的组件,触发 next() }), methods:{ toPage(){ //路由跳转 this.$router.push(xxx) } }, created(){ //获取params this.$route.params //获取query this.$route.query } } </script>
vue3
组合式API
<template> <div> <button @click="toPage">路由跳转</button> </div> </template> <script> import { defineComponent } from 'vue' import { useRoute, useRouter } from 'vue-router' export default defineComponent({ beforeRouteEnter (to, from, next) { // 在渲染该组件的对应路由被 confirm 前调用 next() }, beforeRouteLeave ((to, from, next)=>{//离开当前的组件,触发 next() }), beforeRouteLeave((to, from, next)=>{//离开当前的组件,触发 next() }), setup() { const router = useRouter() const route = useRoute() const toPage = () => { router.push(xxx) } //获取params 注意是route route.params //获取query route.query return { toPage } }, }); </script>
setup语法糖 我之所以用beforeRouteEnter作为路由守卫的示例是因为它在setup语法糖中是无法使用的;大家都知道setup中组件实例已经创建,是能够获取到组件实例的。而beforeRouteEnter是再进入路由前触发的,此时组件还未创建,所以是无法用在setup中的;如果想在setup语法糖中使用则需要再写一个script 如下:
<template> <div> <button @click="toPage">路由跳转</button> </div> </template> <script> export default { beforeRouteEnter(to, from, next) { // 在渲染该组件的对应路由被 confirm 前调用 next() }, }; </script> <script setup> import { useRoute, useRouter,onBeforeRouteLeave, onBeforeRouteUpdate } from 'vue-router' const router = useRouter() const route = useRoute() const toPage = () => { router.push(xxx) } //获取params 注意是route route.params //获取query route.query //路由守卫 onBeforeRouteUpdate((to, from, next)=>{//当前组件路由改变后,进行触发 next() }) onBeforeRouteLeave((to, from, next)=>{//离开当前的组件,触发 next() }) </script>
7、vue3中使用this Vue3 的 setup 中无法使用 this 这个上下文对象。可能刚接触 Vue3 的兄弟会有点懵,我想使用 this 上的属性和方法应该怎么办呢。虽然不推荐这样使用,但依然可以通过 getCurrentInstance 方法获取上下文对象:
<script setup> import { getCurrentInstance } from 'vue' // 以下两种方法都可以获取到上下文对象 const { ctx } = getCurrentInstance() const { proxy } = getCurrentInstance() </script>
注意:ctx 只能在开发环境使用,生成环境为 undefined 。 推荐使用 proxy ,在开发环境和生产环境都可以使用。 8、插槽的使用 在 Vue2 的中一般是通过 slot 属性指定模板的位置,通过 slot-scope 获取作用域插槽的数据,如: vue2
<!-- 父组件 --> <script setup> import ChildView from './ChildView.vue' </script> <template> <div>parent<div> <ChildView> <template slot="content" slot-scope="{ msg }"> <div>{{ msg }}</div> </template> </ChildView> </template> <!-- 子组件 --> <template> <div>child</div> <slot name="content" msg="hello vue3!"></slot> </template>
在 Vue3 中则是通过 v-slot 这个指令来指定模板的位置,同时获取作用域插槽的数据,如
vue3
<!-- 父组件 --> <script setup> import ChildView from './ChildView.vue' </script> <template> <div>parent</div> <ChildView> <template v-slot:content="{ msg }"> <div>{{ msg }}</div> </template> </ChildView> </template> <!-- ChildView 也可以简写为: --> <ChildView> <template #content="{ msg }"> <div>{{ msg }}</div> </template> </ChildView> <!-- 子组件 --> <template> <div>child</div> <slot name="content" msg="hello vue3!"></slot> </template>
注意:v-slot 在 Vue2 中也可以使用,但必须是 Vue2.6+ 的版本。 9、缓存路由组件 缓存一般的动态组件,Vue3 和 Vue2 的用法是一样的,都是使用 KeepAlive 包裹 Component。但缓存路由组件,Vue3 需要结合插槽一起使用:
// Vue2 中缓存路由组件 <KeepAlive> <RouterView /> </KeepAlive> // Vue3 中缓存路由组件 <RouterView v-slot="{ Component }"> <KeepAlive> <Component :is="Component"></Component> </KeepAlive> </RouterView>
一个持续存在的组件可以通过 onActivated() 和 onDeactivated() 两个生命周期钩子注入相应的逻辑:
<script setup> import { onActivated, onDeactivated } from 'vue' onActivated(() => { // 调用时机为首次挂载 // 以及每次从缓存中被重新插入时 }) onDeactivated(() => { // 调用时机为从 DOM 上移除、进入缓存 // 以及组件卸载时 }) </script>
10、逻辑复用
Vue2 中逻辑复用主要是采用 mixin,但 mixin 会使数据来源不明,同时会引起命名冲突。所以 Vue3 更推荐的是全新的 Composition Api。
下面是鼠标跟踪的例子,我们可以把逻辑提取出来:
eg1:
// mouse.js import { ref, onMounted, onUnmounted } from 'vue' // 按照惯例,组合式函数名以 use 开头 export function useMouse() { // 组合式函数管理的数据 const x = ref(0) const y = ref(0) function update(event) { x.value = event.pageX y.value = event.pageY } // 组合式函数可以挂靠在所属组件的生命周期上,来启动和卸载副作用 onMounted(() => window.addEventListener('mousemove', update)) onUnmounted(() => window.removeEventListener('mousemove', update)) // 通过返回值暴露所管理的数据 return { x, y } }
这时候在组件中我们就可以直接使用 mouse.js 暴露的数据了。
<script setup> import { useMouse } from './mouse.js' const { x, y } = useMouse() </script> <template>Mouse position is at: {{ x }}, {{ y }}</template>
eg2:
// useCount.js const useCount = (initValue = 1) => { const count = ref(initValue) const increase = (delta) => { if(typeof delta !== 'undefined'){ count.value += delta }else{ count.value++ } } const multiple = computed(() => count.value * 2) const decrease = (delta) => { if(typeof delta !== 'undefined'){ count.value -= delta }else{ count.value-- } } const reset = () => count.value = initValue return { count, multiple, increase, decrease, reset } } export default useCount
<template> <p>{{count}}</p> <p>{{multiple}}</p> <el-button @click="addCount">count++</el-button> <el-button @click="subCount">count--</el-button> <el-button @click="resetCount">reset</el-button> </template> <script setup> import useCount from "@/hooks/useCount" const {count,multiple,increase,decrease,reset} = useCount(10) const addCount = () => increase() const subCount = () => decrease() const resetCount = () => reset() </script>
11、全局 API
Vue2 中的全局属性或全局方法,是在构造函数 Vue 的原型对象上进行添加,如:Vue.prototype.$axios = axios 。但在 Vue3 中,需要在 app 实例上添加:
vue3
// main.js app.config.globalProperties.$axios = axios // 在组件中使用 <script setup> import { getCurrentInstance } from 'vue' const { proxy } = getCurrentInstance() proxy.$axios.get('http://...') </script>
Vue3 中其他的全局 API,如 directive 、component 等,跟 Vue2 的用法都差不多,只不过一个是在 Vue 上调用,一个是在 app 实例上调用:
// main.js // 全局自定义指令 app.directive('focus', { mounted(el) { el.focus() } }) // 全局自定义组件 import CustomComp from './components/CustomComp.vue' app.component('CustomComp', CustomComp)
注意的是,Vue3 废弃了 filter 这个方法,因为通过函数或 computed 可以实现一样的功能。
12、与 TypeScript 结合使用
与 TypeScript 结合使用,我们只需要在
为 props 标注类型
- 运行时声明。当使用
<script setup lang="ts"> const props = defineProps({ foo: { type: String, required: true }, bar: Number }) props.foo // string props.bar // number | undefined </script>
这被称为 运行时声明 ,因为传递给 defineProps() 的参数会作为运行时的 props 选项使用。
- 基于类型的声明。我们还可以通过泛型参数来定义 props 的类型,这种方式更加常用:
<script setup lang="ts"> interface Props { foo: string bar?: number } const props = defineProps<Props>() </script>
这被称为 基于类型的声明 ,编译器会尽可能地尝试根据类型参数推导出等价的运行时选项。这种方式的不足之处在于,失去了定义 props 默认值的能力。为了解决这个问题,我们可以使用 withDefaults 宏函数:
<script setup lang="ts"> interface Props { msg?: string labels?: string[] } const props = withDefaults(defineProps<Props>(), { msg: 'hello vue3!', labels: () => ['one', 'two'] }) </script>
为 ref() 标注类型
- 默认推导类型。ref 会根据初始化时的值自动推导其类型:
import { ref } from 'vue' const year = ref(2022) year.value = '2022' // TS Error: 不能将类型 string 分配给类型 number
通过接口指定类型。有时我们可能想为 ref 内的值指定一个更复杂的类型,可以使用 Ref 这个接口:
import { ref } from 'vue' import type { Ref } from 'vue' const year: Ref<string | number> = ref('2022') year.value = 2022 // 成功!
通过泛型指定类型。我们也可以在调用 ref() 时传入一个泛型参数,来覆盖默认的推导行为:
const year = ref<string | number>('2022') year.value = 2022 // 成功!
为 reactive() 标注类型
- 默认推导类型。reactive() 也会隐式地从它的参数中推导类型:
import { reactive } from 'vue' const book = reactive({ title: 'Vue 3 指引' }) book.year = 2022 // TS Error: 类型 { title: string; } 上不存在属性 year
通过接口指定类型。要显式地指定一个 reactive 变量的类型,我们可以使用接口:
import { reactive } from 'vue' interface Book { title: string year?: number } const book: Book = reactive({ title: 'Vue 3 指引' }) book.year = 2022 // 成功!
13、Pinia状态管理篇
将复杂逻辑的状态以及修改状态的方法提升到store内部管理,可以避免props的层层传递,减少props复杂度,状态管理更清晰
• 定义一个store(非声明式):User.ts
import { computed, reactive } from 'vue' import { defineStore } from 'pinia' type UserInfo = { userName: string realName: string headImg: string organizationFullName: string } export const useUserStore = defineStore('user', () => { const userInfo = reactive<UserInfo>({ userName: '', realName: '', headImg: '', organizationFullName: '' }) const fullName = computed(() => { return `${userInfo.userName}[${userInfo.realName}]` }) const setUserInfo = (info: UserInfo) => { Object.assgin(userInfo, {...info}) } return { userInfo, fullName, setUserInfo } })
• 在组件中使用
<template> <div class="welcome" font-JDLangZheng> <el-space> <el-avatar :size="60" :src="userInfo.headImg ? userInfo.headImg : avatar"> </el-avatar> <div> <p>你好,{{ userInfo.realName }},欢迎回来</p> <p style="font-size: 14px">{{ userInfo.organizationFullName }}</p> </div> </el-space> </div> </template> <script setup lang="ts"> import { useUserStore } from '@/stores/user' import avatar from '@/assets/avatar.png' const { userInfo } = useUserStore() </script>
14、Teleport
- eleport 瞬移组件,能将我们的元素移动到DOM中vue app之外的其他位置(有时用于页面需要弹框且弹框不影响布局的情况,相对于body进行定位)
<div class="app-container"> <el-button type="primary" @click="showToast">打开弹框</el-button> </div> <teleport to="body"> <div v-if="visible" class="modal_class"> A man who has not climbed the granted wall is not a true man <el-button style="width: 50%; margin-top: 20px" type="primary" @click="closeToast" >关闭弹框</el-button > </div> </teleport> </template> <script setup> import { ref } from "vue"; const visible = ref(false); const showToast = () => { visible.value = true; }; const closeToast = () => { visible.value = false; }; </script> <style scoped> .modal_class { position: absolute; width: 300px; height: 200px; top: 50%; left: 50%; transform: translate(-50%, -50%); border: 1px solid #ccc; display: flex; flex-direction: column; justify-content: center; align-content: center; padding: 30px; } </style>
15、获取DOM
<template> <el-form ref="formRef"></el-form> </template> <script setup> // 1. 变量名和 DOM 上的 ref 属性必须同名,自动形成绑定 const formRef = ref(null) console.log(formRef.value) // 2. 通过当前组件实例来获取DOM元素 const { proxy } = getCurrentInstance() proxy.$refs.formRef.validate((valid) => { ... }) </script>
16、懒加载组件
// Demo.vue <template> <div>异步加载组件的内容</div> </template>
// ErrorComponent.vue <template> <div>Warning:组件加载异常</div> </template>
// LoadingComponent.vue <template> <div>组件正在加载...<div> </template>
<template> <AsyncDemo /> </template> <script setup> import LoadingComponent from './LoadingComponent.vue' import ErrorComponent from './ErrorComponent.vue' const time = (t,callback = () => {}) => { return new Promise((resolve) => { setTimeout(() => { callback() resolve() },t) }) } const AsyncDemo = defineAsyncComponent({ // 要加载的组件 loader:() => { return new Promise((resolve) => { async function(){ await time(3000) const res = await import("./Demo.vue") resolve(res) } }) }, // 加载异步组件时使用的组件 loadingComponent:LoadingComponent, // 加载失败时使用的组件 errorComponent:ErrorComponent, // 加载延迟(在显示loadingComponent之前的延迟),默认200 delay:0, // 超时显示组件错误,默认永不超时 timeout:5000 }) </script>
17、自定义指令
- 全局自定义指令在main.js中定义
- 局部自定义指令在当前组件中定义
// main.js app.directive("focus",{ mounted(el,bingings,vnode,preVnode){ el.focus() } })
<template> <div> <input type="text" v-focus /> </div> </template> <script setup> const vFocus = { mounted:(el) => el.focus() } </script>
18、css补充
样式穿透
- css >>> className,less /deep/ className, scss ::v-deep className
- vue3中css使用::deep(className)
绑定变量
<template> <div class="name">falcon</div> </template> <script setup> const str = ref('#f00') </script> <style lang="scss" scoped> .name{ background-color:v-bind(str) } </style>
免责声明:本站所有文章内容,图片,视频等均是来源于用户投稿和互联网及文摘转载整编而成,不代表本站观点,不承担相关法律责任。其著作权各归其原作者或其出版社所有。如发现本站有涉嫌抄袭侵权/违法违规的内容,侵犯到您的权益,请在线联系站长,一经查实,本站将立刻删除。 本文来自网络,若有侵权,请联系删除,如若转载,请注明出处:https://yundeesoft.com/89823.html