大家好,欢迎来到IT知识分享网。
多线程的使用,本文主要简单介绍使用多线程的几种方式,并使用几个简单的例子来介绍多线程,使用编译器为visual studio。
一、AsyncFuture
使用的知识点有std::async和std::future
1、std::async函数原型
template<class Fn, class... Args>
future<typename result_of<Fn(Args...)>::type> async(launch policy, Fn&& fn, Args&&...args);
功能:第二个参数接收一个可调用对象(仿函数、lambda表达式、类成员函数、普通函数……)作为参数,并且异步或是同步执行他们。
对于是异步执行还是同步执行,由第一个参数的执行策略决定:
(1)std::launch::async 传递的可调用对象异步执行;
(2)std::launch::deferred 传递的可调用对象同步执行;
(3)std::launch::async | std::launch::deferred 可以异步或是同步,取决于操作系统,我们无法控制;
(4)如果不指定具体的策略,则默认执行(3)。
如果选择异步执行策略,调用get时,如果异步执行没有结束,get会阻塞当前调用线程,直到异步执行结束并获得结果,如果异步执行已经结束,不等待获取执行结果;如果选择同步执行策略,只有当调用get函数时,同步调用才真正执行,这也被称为函数调用被延迟。
返回结果std::future的状态:
(1)deffered:异步操作还没有开始;
(2)ready:异步操作已经完成;
(3)timeout:异步操作超时。
例子
#include "stdfax.h"
/*
std::async,std::future
*/
int main()
{
// step1 possibly start thread immediately
std::future<int> ft1(std::async(sum, 1, 11));
std::future<int> ft2(std::async(sum, 1, 101));
try {
// step2 to get result we have to call future.get()
int a = ft1.get();
int b = ft2.get();
cout << a << " + " << b << " = " << a + b << endl;
}
catch (std::exception& e)
{
cout << e.what() << endl;
}
return 0;
}
二、PackageTaks
使用的知识点有std::package_task和std::future
1、std::package_task是一个模板类
std::packaged_task包装任何可调用目标(函数、lambda表达式、bind表达式、函数对象)以便它可以被异步调用。它的返回值或抛出的异常被存储于能通过std::future对象访问的共享状态中。
例子
#include "stdfax.h"
/*
std::package_task, std::promise,std::future
*/
int main()
{
// step 1 does not start thread yet
std::packaged_task<int(int, int)> task1(sum);
std::packaged_task<int(int, int)> task2(sum);
// step2 create future using task
std::future<int> ft1 = task1.get_future();
std::future<int> ft2 = task2.get_future();
// step3 we have to start the thread
// start the task (or thread)
task1(1, 11);
task2(1, 101);
try {
// step 4 now get the result of processing
int a = ft1.get();
int b = ft2.get();
cout << a << " + " << b << " = " << a + b << endl;
}
catch (std::exception& e)
{
cout << e.what() << endl;
}
}
三、PromiseFuture
使用的知识点有std::thread,std::promise和std::future
1、std::future
std::future期待一个返回,从一个异步调用的角度来说,future更像是执行函数的返回值,C++标准库使用std::future为一次性事件建模,如果一个事件需要等待特定的一次性事件,那么这线程可以获取一个future对象来代表这个事件。异步调用往往不知道何时返回,但是如果异步调用的过程需要同步,或者说后一个异步调用需要使用前一个异步调用的结果。这个时候就要用到future。
2、std::promise
promise 对象可以保存某一类型 T 的值,该值可被 future 对象读取(可能在另外一个线程中),因此 promise 也提供了一种线程同步的手段。在 promise 对象构造时可以和一个共享状态(通常是std::future)相关联,并可以在相关联的共享状态(std::future)上保存一个类型为 T 的值。
可以通过 get_future 来获取与该 promise 对象相关联的 future 对象,调用该函数之后,两个对象共享相同的共享状态(shared state)
- promise 对象是异步 Provider,它可以在某一时刻设置共享状态的值。
- future 对象可以异步返回共享状态的值,或者在必要的情况下阻塞调用者并等待共享状态标志变为 ready,然后才能获取共享状态的值。
get_future()
此函数返回一个与 promise 共享状态相关联的 future 。返回的 future 对象可以访问由 promise 对象设置在共享状态上的值或者某个异常对象。只能从 promise 共享状态获取一个 future 对象。在调用该函数之后,promise 对象通常会在某个时间点准备好(设置一个值或者一个异常对象),如果不设置值或者异常,promise 对象在析构时会自动地设置一个 future_error 异常(broken_promise)来设置其自身的准备状态。
例子
#include "stdfax.h"
/*
std:thread,std::promise,std:future
*/
int main()
{
// step 1 create a promise with return type
std::promise<int> prm,prm2;
// step 2 create a future using the promise
std::future<int> ft = prm.get_future();
std::future<int> ft2 = prm2.get_future();
// step 3 create a thread using promise
std::thread thr(sum_prm, std::ref(prm), 1, 11);
std::thread thr2(sum_prm, std::ref(prm2), 1, 101);
// step 4 detach thread
// you should not forget this step
thr.detach();
thr2.detach();
try {
// step 5 now get the result of processing
int a = ft.get();
int b = ft2.get();
cout << a << " + " << b << " = " << a + b << endl;
}
catch (std::exception& e)
{
cout << e.what() << endl;
}
return 0;
}
零、例子的通用代码
stdafx.h
#include <stdio.h>
#include <tchar.h>
#include "thread_inc.h"
stdafx.cpp
#include "stdfax.h"
#include "thread_inc.cpp"
thread_inc.h
#include <iostream>
#include <thread>
#include <memory>
#include <future>
#include <functional>
#include <utility>
#include <exception>
#include <sstream>
using namespace std;
extern int sum(int st, int ed);
extern void sum_prm(std::promise<int>& prm, int st, int ed);
thread_inc.cpp 此文件的代码不参与生成,点击thread_inc.cpp,右键属性,在常规-》项目类型,设置不参与生成。
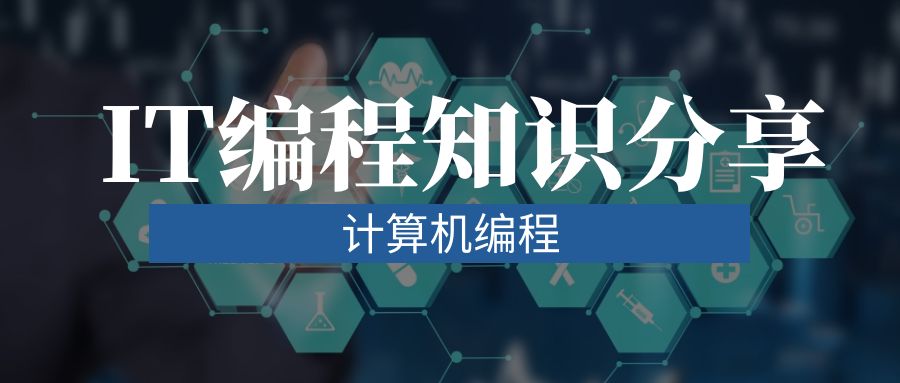
int sum(int st, int ed)
{
int rlt = 0;
for (int i = st; i < ed; ++i)
{
// 设置异常
if (i == (st + ed) / 2)
{
std::ostringstream os;
os << "sum(" <<st<< ", " << ed << ") - throw exception at: " << i;
throw std::runtime_error(os.str().c_str());
}
rlt += i;
}
return rlt;
}
void sum_prm(std::promise<int>& prm, int st, int ed)
{
int rlt = 0;
try {
for (int i = st; i < ed; ++i)
{
// 设置异常
if (i == (st + ed) / 2)
{
std::ostringstream os;
os << "sum_prm(" << st << ", " << ed << ") - throw exception at: " << i;
throw std::runtime_error(os.str().c_str());
}
rlt += i;
}
// prm.set_value(rlt);
prm.set_value_at_thread_exit(rlt);
}
catch (std::exception& e)
{
//prm.set_exception(std::current_exception() )
prm.set_exception_at_thread_exit(std::current_exception());
}
}
免责声明:本站所有文章内容,图片,视频等均是来源于用户投稿和互联网及文摘转载整编而成,不代表本站观点,不承担相关法律责任。其著作权各归其原作者或其出版社所有。如发现本站有涉嫌抄袭侵权/违法违规的内容,侵犯到您的权益,请在线联系站长,一经查实,本站将立刻删除。 本文来自网络,若有侵权,请联系删除,如若转载,请注明出处:https://yundeesoft.com/51169.html