大家好,欢迎来到IT知识分享网。
需求:
linux系统支持4g网络,但是没有统计流量的工具,只能通过第三方平台查看流量使用情况,由于需要统计某个时间段内流量消耗,就需每隔2分钟就查询下流量消耗情况
代码1(获取流量数据):
import requests
import json
import time
import base64
import xlwt
import xlrd
from xlutils.copy import copy
import os
#使用账号密码登录
def login(url,username,password,code,token):
url = "%s/api/user/login"%url
register_data = {"username": username,
"password": password,
"token": token,
"captcha": code}
response = requests.post(url, json=register_data).json()
#print (json.dumps(response,indent=4,ensure_ascii=False))
msg = response["msg"]
if msg == "令牌生成成功":
#print ("登录成功")
channelCustId = response["data"]["channelCustId"]
accessToken = response["data"]["accessToken"]
return channelCustId,accessToken
else:
print (msg)
return False,False
def check(url,channelCustId,Authorization,paramNum):
url = "%s/api/querySimCard"%url
header = {"authorization":Authorization}
register_data = {"channelCustId": channelCustId,
"current": 1,
"pageSize": 10,
"paramNum": paramNum}
response = requests.post(url,headers = header ,json=register_data).json()
#print (json.dumps(response,indent=4,ensure_ascii=False))
success = response['success']
stateCode = response["data"]["list"][0]["stateCode"]
useTotal = response["data"]["list"][0]["useTotal"]
#print (success,paramNum,stateCode,useTotal)
times = time.strftime('%Y-%m-%d %H:%M:%S',time.localtime())
test_list = [paramNum,stateCode,times,useTotal]
return test_list
def write_excel_append(file_name,value):
if os.path.exists('%s'%file_name):
pass
#print ("文件已存在")
else:
#print ("文件不存在,新建excel")
#新建excel文件
add_excel = xlwt.Workbook()
#新建sheet名
excel_sheet_name = 'result'
sheet = add_excel.add_sheet(excel_sheet_name)
add_excel.save(file_name)
try:
#获取需要写入数据的行数
index = len(value)
#print ("写入数据的行数:%s"%index)
# 获取工作簿中的所有表格
work_book = xlrd.open_workbook(file_name) # 打开工作簿
all_sheets = work_book.sheet_names()
#print (all_sheets)
# 获取表格中已存在的数据的行数
work_sheet = work_book.sheet_by_name(all_sheets[0]) # 获取工作簿中所有表格中的的第一个表格
rows_old = work_sheet.nrows
#print ("表格中已存在的数据的行数%s"%rows_old)
# 将xlrd对象拷贝转化为xlwt对象
new_work_book = copy(work_book)
new_work_sheet = new_work_book.get_sheet(0) # 获取转化后工作簿中的第一个表格
for i in range(0, index):
for j in range(0, len(value[i])):
new_work_sheet.write(i+rows_old, j, value[i][j]) # 追加写入数据
new_work_book.save(file_name) # 保存工作簿
#print("xls格式表格【追加】写入数据成功!")
except Exception as e:
print ('写入数据失败:',e)
if __name__ == '__main__':
url = "https://xxx.xxx.com"
username = "账号"
password = "密码"
#SIM 卡序号
paramNum = "sim卡ICCID号码"
#4g测试结果保存文件
excel_file_name = '4GTest_Result.xlsx'
while True:
token = "测试数据"
code = "测试数据"
channelCustId,Authorization = login(url,username,password,code,token)
if channelCustId == False:
print ("登录失败")
time.sleep(2)
else:
print ("登录成功")
break
tabal_title = [["识别记录数","ICCID","状态","查询流量时间","已用流量"]]
write_excel_append(excel_file_name,tabal_title)
while True:
all_num = check_num()# 该方法已移除,请忽略
print ("获取流量数据")
tabal_content = check(url,channelCustId,Authorization,paramNum)
print (tabal_content)
print ("将数据写入excel")
write_excel_append(excel_file_name,[[all_num]+tabal_content])
print ("2分钟后继续获取")
time.sleep(120)
代码2(生成流量曲线图):
import xlsxwriter
import xlrd
def excel(excel_name,sheet_name,Chart_excel):
# 创建一个excel
workbook = xlsxwriter.Workbook(Chart_excel)
# 创建一个sheet
worksheet = workbook.add_worksheet(sheet_name)
# 写入数据
headings = ['time', '4G', 'num']
worksheet.write_row('A1', headings)
data = xlrd.open_workbook(excel_name)
table = data.sheets()[0]
x_list = table.col_values(3)#读取时间
x_list.remove(x_list[0])
#print (x_list)
y_list = table.col_values(4)#读取流量值
y_list.remove(y_list[0])
#print (y_list)
y_list1 = [float(x) for x in y_list]#
worksheet.write_column('A2', x_list)
worksheet.write_column('B2', y_list1)
# 创建一个折线图(line chart)
line_chart = workbook.add_chart({'type': 'line'})
time_result = 10
# 配置第一个系列数据
line_chart.add_series({
'name': '=%s!$B$1'%sheet_name,
'categories': '=%s!$A$2:$A$%s'%(sheet_name,len(x_list)), #例如“A2至A7”作为图表数据标签(X轴)
'values': '=%s!$B$2:$B$%s'%(sheet_name,len(x_list)), #例如“B2至B7”作为图表数据标签(Y轴)
'line': {'color': 'red'},
})
# 设置图表的title 和 x,y轴信息
line_chart.set_title({'name': '4G已使用流量曲线'})
line_chart.set_x_axis({'name': '时间(m/d H:M:S)'})
line_chart.set_y_axis({'name': '单位(M)'})
# 把图表插入到worksheet并设置偏移,及图表大小
worksheet.insert_chart('D4', line_chart, {'x_scale': 2, 'y_scale': 1.5})
workbook.close()
if __name__ == '__main__':
excel_name = "4GTest_Result.xlsx"
Chart_excel = "Line_Chart.xlsx"
sheet_name = "result"
excel(excel_name,sheet_name,Chart_excel)
执行(第一段代码):
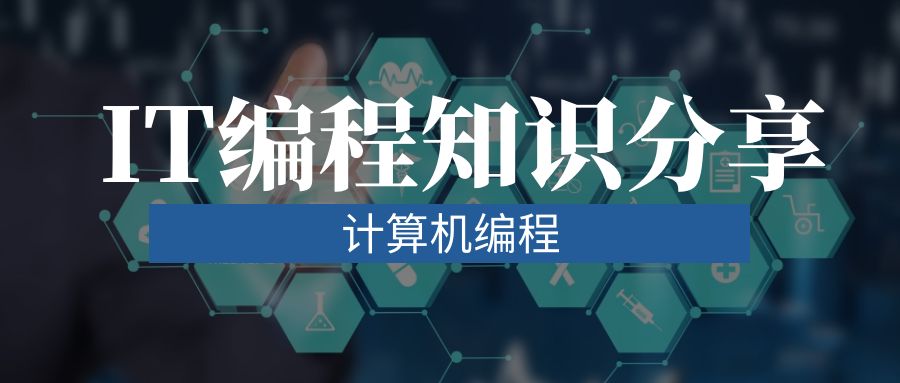
执行(第二段代码):
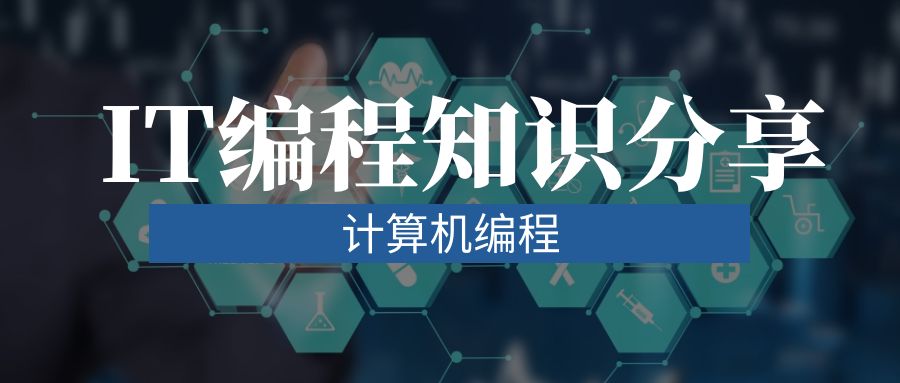
测试结果:
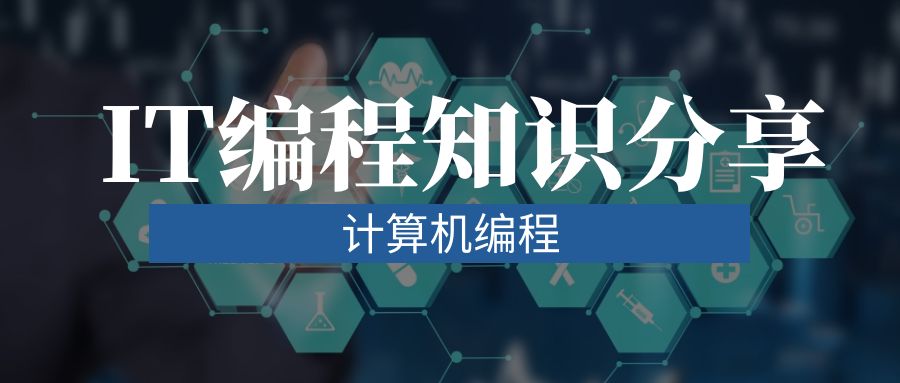
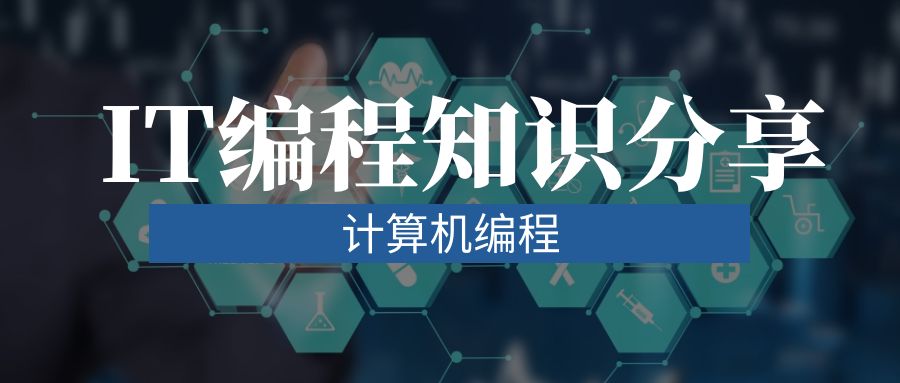
免责声明:本站所有文章内容,图片,视频等均是来源于用户投稿和互联网及文摘转载整编而成,不代表本站观点,不承担相关法律责任。其著作权各归其原作者或其出版社所有。如发现本站有涉嫌抄袭侵权/违法违规的内容,侵犯到您的权益,请在线联系站长,一经查实,本站将立刻删除。 本文来自网络,若有侵权,请联系删除,如若转载,请注明出处:https://yundeesoft.com/67085.html