大家好,欢迎来到IT知识分享网。
26 – 27 – 箭头函数:基础 & this 关键字
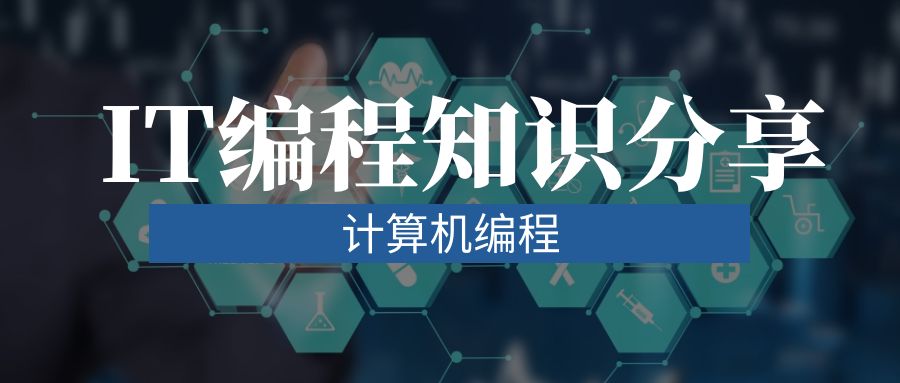
原文:https://dev.to/bhagatparwinder/arrow-function-basics-34cm
简介
箭头函数是在 ES6 引入的,相对于函数表达式来说是一种更简洁的方式。
箭头函数名称的来源是因为使用了 =>。
语法
const functionName = (arg1, arg2, ... argN) => { return value; }
例子
const multiply = (a, b) => { return a * b; } console.log(multiply(7, 8)); // 56 console.log(multiply(3, 2)); // 6
关键特点
- • 箭头函数类似匿名函数
- • 若只有一个参数,可以省略小括号
const square = x => { return x * x; } console.log(square(2)); // 4 console.log(square(7)); // 49
这个情形的唯一陷阱是当只有一个参数且需要解构时:
const foo = ({name = "New User"}) => name; console.log(foo({})); // New User console.log(foo({name: "Parwinder"})); // Parwinder
- • 若没有参数,需要添加上小括号
const greeting = () => { return "Hello World!"; } console.log(greeting()); // Hello World!
- • 若函数体是一个表达式且只返回该表达式,我们可以移出小括号和 return 关键字。
const greeting = () => "Hello World!"; console.log(greeting()); // Hello World
现在我们知道了所有的关键特点,让我们来重写获取正方形的面积:
const square = x => x * x; console.log(square(4)); // 16
this 关键字
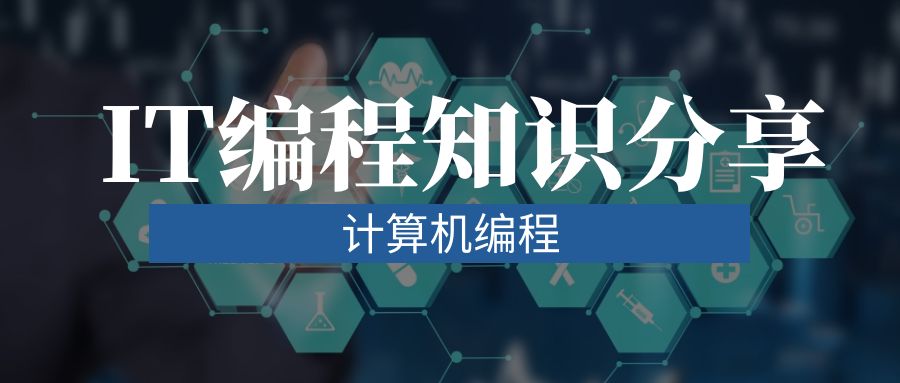
this 关键字和函数
JavaScript 中的 this 关键字是执行上下文的一个属性,它可能是全局的、函数内的或者是 eval 中的。对于普通的函数,this 会根据调用它方式不同而变化。
- 1. 函数直接调用时,this 指向全局对象;
- 2. 用在构造函数中,this 代表一个新对象;
- 3. 当函数作为一个对象的方法被调用,this 就代表那个对象;
- 4. 在严格模式下, this 的值为 undefined;
- 5. 在事件中,this 指向接收到事件的元素;
我们使用这种行为已经很久了,以至于大多数JavaScript开发者都已经习惯了。
函数直接调用,this 代表全局对象
function foo() { return this; }; console.log(foo()); // window object in a browser, global object for node execution
用在构造函数中,this 代表一个新对象
function Order(main, side, dessert) { this.main = main; this.side = side; this.dessert = dessert; this.order = function () { return `I will have ${this.main} with ${this.side} and finish off with a ${this.dessert}`; } } const newOrder = new Order("sushi", "soup", "yogurt"); console.log(newOrder.order()); // I will have sushi with soup and finish off with a yogurt
当函数作为一个对象的方法被调用,this 就代表那个对象
const myObject = { main: "butter chicken", side: "rice", dessert: "ice cream", order: function () { return `I will have ${this.main} with ${this.side} and finish off with ${this.dessert}`; } } console.log(myObject.order()); // I will have butter chicken with rice and finish off with ice cream
上面的例子中,this 指向 myObject,可以获取它上面的属性。
在严格模式下, this 的值为 undefined
"use strict"; function foo() { return this; }; console.log(foo() === undefined); // true
在事件中,this 指向接收到事件的元素
<!DOCTYPE html>
<html lang="en">
<head>
<meta content="text/html;charset=utf-8" http-equiv="Content-Type">
<meta content="utf-8" http-equiv="encoding">
</head>
<body>
<button id="mybutton">
Click me!
</button>
<script>
var element = document.querySelector("#mybutton");
element.addEventListener('click', function (event) {
console.log(this); // Refers to the button clicked
console.log(this.id); // mybutton
}, false);
</script>
</body>
</html>
免责声明:本站所有文章内容,图片,视频等均是来源于用户投稿和互联网及文摘转载整编而成,不代表本站观点,不承担相关法律责任。其著作权各归其原作者或其出版社所有。如发现本站有涉嫌抄袭侵权/违法违规的内容,侵犯到您的权益,请在线联系站长,一经查实,本站将立刻删除。 本文来自网络,若有侵权,请联系删除,如若转载,请注明出处:https://yundeesoft.com/77981.html